Commit a8a1ef7
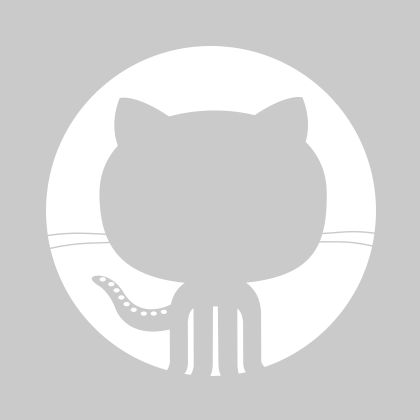
telwertowski
·
1 parent 701011c commit a8a1ef7
File tree
19 files changed
+259
-409
lines changed- src
- gui
- plugins
- grass
- scale_bar
- ui
19 files changed
+259
-409
lines changedLines changed: 4 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
76 | 76 |
| |
77 | 77 |
| |
78 | 78 |
| |
| 79 | + | |
79 | 80 |
| |
80 | 81 |
| |
81 |
| - | |
82 | 82 |
| |
| 83 | + | |
83 | 84 |
| |
84 | 85 |
| |
85 | 86 |
| |
| |||
199 | 200 |
| |
200 | 201 |
| |
201 | 202 |
| |
202 |
| - | |
| 203 | + | |
203 | 204 |
| |
204 | 205 |
| |
205 | 206 |
| |
206 | 207 |
| |
207 | 208 |
| |
208 | 209 |
| |
| 210 | + | |
209 | 211 |
| |
210 | 212 |
| |
211 | 213 |
| |
|
Lines changed: 55 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + |
Lines changed: 37 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + |
Lines changed: 17 additions & 38 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
65 | 65 |
| |
66 | 66 |
| |
67 | 67 |
| |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 | 68 |
| |
76 | 69 |
| |
77 | 70 |
| |
| |||
98 | 91 |
| |
99 | 92 |
| |
100 | 93 |
| |
101 |
| - | |
102 |
| - | |
103 |
| - | |
104 |
| - | |
105 |
| - | |
106 |
| - | |
107 |
| - | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
108 | 97 |
| |
109 | 98 |
| |
110 |
| - | |
111 |
| - | |
112 |
| - | |
113 |
| - | |
114 |
| - | |
115 |
| - | |
116 |
| - | |
117 |
| - | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
118 | 103 |
| |
119 | 104 |
| |
120 | 105 |
| |
| |||
133 | 118 |
| |
134 | 119 |
| |
135 | 120 |
| |
136 |
| - | |
137 |
| - | |
| 121 | + | |
| 122 | + | |
138 | 123 |
| |
139 | 124 |
| |
140 | 125 |
| |
| |||
189 | 174 |
| |
190 | 175 |
| |
191 | 176 |
| |
192 |
| - | |
| 177 | + | |
193 | 178 |
| |
194 | 179 |
| |
195 | 180 |
| |
196 |
| - | |
| 181 | + | |
197 | 182 |
| |
198 | 183 |
| |
199 | 184 |
| |
200 | 185 |
| |
201 | 186 |
| |
202 | 187 |
| |
203 |
| - | |
| 188 | + | |
204 | 189 |
| |
205 | 190 |
| |
206 | 191 |
| |
207 |
| - | |
| 192 | + | |
208 | 193 |
| |
209 | 194 |
| |
210 | 195 |
| |
| |||
222 | 207 |
| |
223 | 208 |
| |
224 | 209 |
| |
225 |
| - | |
| 210 | + | |
226 | 211 |
| |
227 | 212 |
| |
228 |
| - | |
229 |
| - | |
230 |
| - | |
231 |
| - | |
| 213 | + | |
232 | 214 |
| |
233 | 215 |
| |
234 | 216 |
| |
235 | 217 |
| |
236 | 218 |
| |
237 | 219 |
| |
238 |
| - | |
| 220 | + | |
239 | 221 |
| |
240 | 222 |
| |
241 |
| - | |
242 |
| - | |
243 |
| - | |
244 |
| - | |
| 223 | + | |
245 | 224 |
| |
246 | 225 |
| |
247 | 226 |
| |
|
Lines changed: 0 additions & 9 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
23 | 23 |
| |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 | 26 |
| |
31 | 27 |
| |
32 | 28 |
| |
| |||
56 | 52 |
| |
57 | 53 |
| |
58 | 54 |
| |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 | 55 |
| |
65 | 56 |
| |
66 | 57 |
|
Lines changed: 8 additions & 42 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
115 | 115 |
| |
116 | 116 |
| |
117 | 117 |
| |
118 |
| - | |
119 |
| - | |
120 |
| - | |
121 |
| - | |
122 |
| - | |
123 |
| - | |
124 |
| - | |
125 |
| - | |
| 118 | + | |
126 | 119 |
| |
127 | 120 |
| |
128 | 121 |
| |
129 | 122 |
| |
130 | 123 |
| |
131 |
| - | |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 |
| - | |
136 |
| - | |
137 |
| - | |
138 |
| - | |
| 124 | + | |
139 | 125 |
| |
140 | 126 |
| |
141 | 127 |
| |
| |||
150 | 136 |
| |
151 | 137 |
| |
152 | 138 |
| |
153 |
| - | |
154 |
| - | |
155 |
| - | |
156 |
| - | |
157 |
| - | |
| 139 | + | |
158 | 140 |
| |
159 | 141 |
| |
160 |
| - | |
161 |
| - | |
162 |
| - | |
163 |
| - | |
| 142 | + | |
164 | 143 |
| |
165 | 144 |
| |
166 | 145 |
| |
167 | 146 |
| |
168 | 147 |
| |
169 |
| - | |
170 |
| - | |
171 |
| - | |
172 |
| - | |
173 |
| - | |
| 148 | + | |
174 | 149 |
| |
175 | 150 |
| |
176 |
| - | |
177 |
| - | |
178 |
| - | |
179 |
| - | |
| 151 | + | |
180 | 152 |
| |
181 | 153 |
| |
182 | 154 |
| |
| |||
231 | 203 |
| |
232 | 204 |
| |
233 | 205 |
| |
234 |
| - | |
235 |
| - | |
236 |
| - | |
237 |
| - | |
| 206 | + | |
238 | 207 |
| |
239 | 208 |
| |
240 | 209 |
| |
241 | 210 |
| |
242 | 211 |
| |
243 |
| - | |
244 |
| - | |
245 |
| - | |
246 |
| - | |
| 212 | + | |
247 | 213 |
| |
248 | 214 |
| |
249 | 215 |
| |
|
Lines changed: 0 additions & 10 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 | 25 |
| |
31 | 26 |
| |
32 | 27 |
| |
| |||
91 | 86 |
| |
92 | 87 |
| |
93 | 88 |
| |
94 |
| - | |
95 |
| - | |
96 |
| - | |
97 |
| - | |
98 |
| - | |
99 | 89 |
| |
100 | 90 |
| |
101 | 91 |
|
Lines changed: 12 additions & 64 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
106 | 106 |
| |
107 | 107 |
| |
108 | 108 |
| |
109 |
| - | |
110 |
| - | |
111 |
| - | |
112 |
| - | |
113 |
| - | |
114 |
| - | |
115 |
| - | |
116 |
| - | |
| 109 | + | |
117 | 110 |
| |
118 | 111 |
| |
119 | 112 |
| |
120 | 113 |
| |
121 | 114 |
| |
122 | 115 |
| |
123 | 116 |
| |
124 |
| - | |
125 |
| - | |
126 |
| - | |
127 |
| - | |
128 |
| - | |
129 |
| - | |
130 |
| - | |
131 |
| - | |
| 117 | + | |
132 | 118 |
| |
133 | 119 |
| |
134 | 120 |
| |
135 | 121 |
| |
136 | 122 |
| |
137 | 123 |
| |
138 |
| - | |
139 |
| - | |
140 |
| - | |
141 |
| - | |
142 |
| - | |
143 |
| - | |
144 |
| - | |
145 |
| - | |
| 124 | + | |
146 | 125 |
| |
147 | 126 |
| |
148 | 127 |
| |
| |||
287 | 266 |
| |
288 | 267 |
| |
289 | 268 |
| |
290 |
| - | |
291 |
| - | |
292 |
| - | |
293 |
| - | |
| 269 | + | |
294 | 270 |
| |
295 | 271 |
| |
296 | 272 |
| |
297 | 273 |
| |
298 | 274 |
| |
299 |
| - | |
300 |
| - | |
301 |
| - | |
302 |
| - | |
| 275 | + | |
303 | 276 |
| |
304 | 277 |
| |
305 | 278 |
| |
306 | 279 |
| |
307 | 280 |
| |
308 | 281 |
| |
309 |
| - | |
310 |
| - | |
311 |
| - | |
312 |
| - | |
| 282 | + | |
313 | 283 |
| |
314 | 284 |
| |
315 | 285 |
| |
| |||
336 | 306 |
| |
337 | 307 |
| |
338 | 308 |
| |
339 |
| - | |
340 |
| - | |
341 |
| - | |
342 |
| - | |
343 |
| - | |
| 309 | + | |
344 | 310 |
| |
345 | 311 |
| |
346 |
| - | |
347 |
| - | |
348 |
| - | |
349 |
| - | |
350 |
| - | |
| 312 | + | |
351 | 313 |
| |
352 | 314 |
| |
353 | 315 |
| |
354 | 316 |
| |
355 | 317 |
| |
356 |
| - | |
357 |
| - | |
358 |
| - | |
359 |
| - | |
360 |
| - | |
| 318 | + | |
361 | 319 |
| |
362 | 320 |
| |
363 |
| - | |
364 |
| - | |
365 |
| - | |
366 |
| - | |
| 321 | + | |
367 | 322 |
| |
368 | 323 |
| |
369 | 324 |
| |
370 | 325 |
| |
371 | 326 |
| |
372 |
| - | |
373 |
| - | |
374 |
| - | |
375 |
| - | |
376 |
| - | |
| 327 | + | |
377 | 328 |
| |
378 | 329 |
| |
379 |
| - | |
380 |
| - | |
381 |
| - | |
382 |
| - | |
| 330 | + | |
383 | 331 |
| |
384 | 332 |
| |
385 | 333 |
| |
|
Lines changed: 0 additions & 10 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 | 25 |
| |
30 | 26 |
| |
31 | 27 |
| |
| |||
121 | 117 |
| |
122 | 118 |
| |
123 | 119 |
| |
124 |
| - | |
125 |
| - | |
126 |
| - | |
127 |
| - | |
128 |
| - | |
129 |
| - | |
130 | 120 |
|
Lines changed: 13 additions & 45 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 | 36 |
| |
42 | 37 |
| |
43 | 38 |
| |
44 | 39 |
| |
45 | 40 |
| |
46 | 41 |
| |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 | 42 |
| |
54 | 43 |
| |
55 | 44 |
| |
| |||
132 | 121 |
| |
133 | 122 |
| |
134 | 123 |
| |
135 |
| - | |
136 |
| - | |
137 | 124 |
| |
138 | 125 |
| |
139 | 126 |
| |
| |||
193 | 180 |
| |
194 | 181 |
| |
195 | 182 |
| |
196 |
| - | |
| 183 | + | |
197 | 184 |
| |
198 | 185 |
| |
199 |
| - | |
200 |
| - | |
201 |
| - | |
202 |
| - | |
| 186 | + | |
203 | 187 |
| |
204 | 188 |
| |
205 | 189 |
| |
| |||
208 | 192 |
| |
209 | 193 |
| |
210 | 194 |
| |
211 |
| - | |
| 195 | + | |
212 | 196 |
| |
213 | 197 |
| |
214 |
| - | |
215 |
| - | |
216 |
| - | |
217 |
| - | |
| 198 | + | |
218 | 199 |
| |
219 | 200 |
| |
220 | 201 |
| |
| |||
224 | 205 |
| |
225 | 206 |
| |
226 | 207 |
| |
227 |
| - | |
| 208 | + | |
228 | 209 |
| |
229 |
| - | |
| 210 | + | |
230 | 211 |
| |
231 | 212 |
| |
232 | 213 |
| |
| |||
334 | 315 |
| |
335 | 316 |
| |
336 | 317 |
| |
337 |
| - | |
| 318 | + | |
338 | 319 |
| |
339 | 320 |
| |
340 | 321 |
| |
| |||
348 | 329 |
| |
349 | 330 |
| |
350 | 331 |
| |
| 332 | + | |
351 | 333 |
| |
352 |
| - | |
353 |
| - | |
354 |
| - | |
355 |
| - | |
356 |
| - | |
357 |
| - | |
358 |
| - | |
359 |
| - | |
360 |
| - | |
| 334 | + | |
361 | 335 |
| |
362 | 336 |
| |
363 | 337 |
| |
| |||
444 | 418 |
| |
445 | 419 |
| |
446 | 420 |
| |
447 |
| - | |
448 |
| - | |
449 |
| - | |
450 |
| - | |
| 421 | + | |
451 | 422 |
| |
452 | 423 |
| |
453 | 424 |
| |
| |||
469 | 440 |
| |
470 | 441 |
| |
471 | 442 |
| |
472 |
| - | |
473 |
| - | |
474 |
| - | |
475 |
| - | |
| 443 | + | |
476 | 444 |
| |
477 | 445 |
| |
478 | 446 |
| |
| |||
521 | 489 |
| |
522 | 490 |
| |
523 | 491 |
| |
524 |
| - | |
| 492 | + | |
525 | 493 |
| |
526 | 494 |
| |
527 | 495 |
| |
| |||
548 | 516 |
| |
549 | 517 |
| |
550 | 518 |
| |
551 |
| - | |
| 519 | + | |
552 | 520 |
| |
553 | 521 |
| |
554 | 522 |
| |
|
Lines changed: 0 additions & 10 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 | 25 |
| |
30 | 26 |
| |
31 | 27 |
| |
| |||
74 | 70 |
| |
75 | 71 |
| |
76 | 72 |
| |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 |
| - | |
82 |
| - | |
83 | 73 |
| |
84 | 74 |
| |
85 | 75 |
| |
|
Lines changed: 7 additions & 10 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
36 | 36 |
| |
37 | 37 |
| |
38 | 38 |
| |
39 |
| - | |
40 | 39 |
| |
41 | 40 |
| |
42 | 41 |
| |
| |||
223 | 222 |
| |
224 | 223 |
| |
225 | 224 |
| |
226 |
| - | |
227 |
| - | |
228 |
| - | |
| 225 | + | |
229 | 226 |
| |
230 | 227 |
| |
231 | 228 |
| |
| |||
241 | 238 |
| |
242 | 239 |
| |
243 | 240 |
| |
| 241 | + | |
| 242 | + | |
| 243 | + | |
244 | 244 |
| |
245 |
| - | |
246 |
| - | |
247 |
| - | |
248 |
| - | |
249 |
| - | |
250 |
| - | |
| 245 | + | |
| 246 | + | |
| 247 | + | |
251 | 248 |
| |
252 | 249 |
| |
253 | 250 |
| |
|
Lines changed: 9 additions & 22 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 | 2 |
| |
6 | 3 |
| |
7 | 4 |
| |
| |||
369 | 366 |
| |
370 | 367 |
| |
371 | 368 |
| |
372 |
| - | |
373 |
| - | |
374 |
| - | |
375 |
| - | |
376 |
| - | |
377 |
| - | |
378 |
| - | |
379 |
| - | |
380 |
| - | |
| 369 | + | |
381 | 370 |
| |
382 | 371 |
| |
383 | 372 |
| |
384 | 373 |
| |
385 | 374 |
| |
386 | 375 |
| |
387 |
| - | |
388 |
| - | |
389 |
| - | |
390 |
| - | |
391 |
| - | |
392 |
| - | |
393 | 376 |
| |
394 | 377 |
| |
395 | 378 |
| |
| |||
437 | 420 |
| |
438 | 421 |
| |
439 | 422 |
| |
440 |
| - | |
| 423 | + | |
441 | 424 |
| |
442 | 425 |
| |
443 | 426 |
| |
444 | 427 |
| |
445 | 428 |
| |
446 |
| - | |
| 429 | + | |
447 | 430 |
| |
448 | 431 |
| |
449 | 432 |
| |
| |||
531 | 514 |
| |
532 | 515 |
| |
533 | 516 |
| |
534 |
| - | |
| 517 | + | |
535 | 518 |
| |
536 | 519 |
| |
537 |
| - | |
| 520 | + | |
| 521 | + | |
| 522 | + | |
| 523 | + | |
| 524 | + | |
538 | 525 |
| |
539 | 526 |
| |
540 | 527 |
| |
|
Lines changed: 3 additions & 6 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
44 |
| - | |
| 44 | + | |
45 | 45 |
| |
46 | 46 |
| |
47 | 47 |
| |
48 | 48 |
| |
49 | 49 |
| |
50 |
| - | |
51 |
| - | |
| 50 | + | |
52 | 51 |
| |
53 | 52 |
| |
54 | 53 |
| |
| |||
96 | 95 |
| |
97 | 96 |
| |
98 | 97 |
| |
99 |
| - | |
100 |
| - | |
101 |
| - | |
| 98 | + | |
102 | 99 |
|
Lines changed: 24 additions & 40 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 | 2 |
| |
6 | 3 |
| |
7 | 4 |
| |
| |||
115 | 112 |
| |
116 | 113 |
| |
117 | 114 |
| |
118 |
| - | |
119 |
| - | |
120 |
| - | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
121 | 123 |
| |
122 |
| - | |
123 |
| - | |
| 124 | + | |
| 125 | + | |
124 | 126 |
| |
125 |
| - | |
126 |
| - | |
127 |
| - | |
128 |
| - | |
129 |
| - | |
130 |
| - | |
131 |
| - | |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 |
| - | |
136 |
| - | |
137 |
| - | |
138 |
| - | |
139 |
| - | |
140 |
| - | |
141 |
| - | |
142 |
| - | |
143 |
| - | |
144 |
| - | |
145 |
| - | |
146 |
| - | |
147 |
| - | |
148 |
| - | |
149 |
| - | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
150 | 134 |
| |
151 | 135 |
| |
152 | 136 |
| |
| |||
271 | 255 |
| |
272 | 256 |
| |
273 | 257 |
| |
274 |
| - | |
275 |
| - | |
276 |
| - | |
277 |
| - | |
278 |
| - | |
279 |
| - | |
280 | 258 |
| |
281 | 259 |
| |
282 | 260 |
| |
| |||
381 | 359 |
| |
382 | 360 |
| |
383 | 361 |
| |
384 |
| - | |
| 362 | + | |
| 363 | + | |
| 364 | + | |
| 365 | + | |
| 366 | + | |
| 367 | + | |
| 368 | + | |
385 | 369 |
| |
386 | 370 |
| |
387 | 371 |
| |
|
Lines changed: 12 additions & 26 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 | 2 |
| |
6 | 3 |
| |
7 | 4 |
| |
8 | 5 |
| |
9 | 6 |
| |
10 | 7 |
| |
11 |
| - | |
| 8 | + | |
12 | 9 |
| |
13 | 10 |
| |
14 | 11 |
| |
| |||
81 | 78 |
| |
82 | 79 |
| |
83 | 80 |
| |
84 |
| - | |
| 81 | + | |
85 | 82 |
| |
86 | 83 |
| |
87 | 84 |
| |
88 |
| - | |
| 85 | + | |
89 | 86 |
| |
90 | 87 |
| |
91 |
| - | |
92 |
| - | |
93 |
| - | |
94 |
| - | |
95 |
| - | |
96 |
| - | |
97 |
| - | |
98 |
| - | |
99 |
| - | |
100 | 88 |
| |
101 | 89 |
| |
102 | 90 |
| |
| |||
116 | 104 |
| |
117 | 105 |
| |
118 | 106 |
| |
119 |
| - | |
| 107 | + | |
120 | 108 |
| |
121 | 109 |
| |
122 | 110 |
| |
123 |
| - | |
| 111 | + | |
124 | 112 |
| |
125 | 113 |
| |
126 |
| - | |
127 |
| - | |
128 |
| - | |
129 |
| - | |
130 |
| - | |
131 |
| - | |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 | 114 |
| |
136 | 115 |
| |
137 | 116 |
| |
| |||
148 | 127 |
| |
149 | 128 |
| |
150 | 129 |
| |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
| 136 | + | |
151 | 137 |
| |
152 | 138 |
| |
153 | 139 |
|
Lines changed: 12 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
| 8 | + | |
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
| |||
154 | 154 |
| |
155 | 155 |
| |
156 | 156 |
| |
157 |
| - | |
| 157 | + | |
158 | 158 |
| |
159 | 159 |
| |
160 | 160 |
| |
161 |
| - | |
| 161 | + | |
162 | 162 |
| |
163 | 163 |
| |
164 | 164 |
| |
| |||
190 | 190 |
| |
191 | 191 |
| |
192 | 192 |
| |
193 |
| - | |
| 193 | + | |
194 | 194 |
| |
195 | 195 |
| |
196 | 196 |
| |
197 |
| - | |
| 197 | + | |
198 | 198 |
| |
199 | 199 |
| |
200 | 200 |
| |
| |||
797 | 797 |
| |
798 | 798 |
| |
799 | 799 |
| |
| 800 | + | |
| 801 | + | |
| 802 | + | |
| 803 | + | |
| 804 | + | |
| 805 | + | |
| 806 | + | |
800 | 807 |
| |
801 | 808 |
| |
802 | 809 |
| |
|
Lines changed: 34 additions & 45 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 | 2 |
| |
6 | 3 |
| |
7 | 4 |
| |
8 | 5 |
| |
9 | 6 |
| |
10 | 7 |
| |
11 |
| - | |
| 8 | + | |
12 | 9 |
| |
13 | 10 |
| |
14 | 11 |
| |
| |||
58 | 55 |
| |
59 | 56 |
| |
60 | 57 |
| |
| 58 | + | |
| 59 | + | |
| 60 | + | |
61 | 61 |
| |
62 | 62 |
| |
63 | 63 |
| |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 | 64 |
| |
68 | 65 |
| |
69 | 66 |
| |
| |||
227 | 224 |
| |
228 | 225 |
| |
229 | 226 |
| |
230 |
| - | |
231 |
| - | |
232 |
| - | |
233 | 227 |
| |
234 | 228 |
| |
235 | 229 |
| |
| 230 | + | |
| 231 | + | |
| 232 | + | |
236 | 233 |
| |
237 | 234 |
| |
238 | 235 |
| |
239 |
| - | |
240 |
| - | |
241 |
| - | |
242 |
| - | |
243 |
| - | |
244 |
| - | |
245 |
| - | |
246 |
| - | |
247 |
| - | |
| 236 | + | |
248 | 237 |
| |
249 | 238 |
| |
250 | 239 |
| |
251 |
| - | |
| 240 | + | |
252 | 241 |
| |
253 | 242 |
| |
254 | 243 |
| |
| |||
315 | 304 |
| |
316 | 305 |
| |
317 | 306 |
| |
318 |
| - | |
319 |
| - | |
320 |
| - | |
321 |
| - | |
322 |
| - | |
323 |
| - | |
324 |
| - | |
325 |
| - | |
326 |
| - | |
| 307 | + | |
327 | 308 |
| |
328 | 309 |
| |
329 |
| - | |
330 |
| - | |
| 310 | + | |
| 311 | + | |
331 | 312 |
| |
332 | 313 |
| |
333 | 314 |
| |
| |||
341 | 322 |
| |
342 | 323 |
| |
343 | 324 |
| |
344 |
| - | |
| 325 | + | |
345 | 326 |
| |
346 | 327 |
| |
347 | 328 |
| |
| |||
362 | 343 |
| |
363 | 344 |
| |
364 | 345 |
| |
365 |
| - | |
366 |
| - | |
367 |
| - | |
368 |
| - | |
369 |
| - | |
370 |
| - | |
371 |
| - | |
372 |
| - | |
373 |
| - | |
| 346 | + | |
374 | 347 |
| |
375 | 348 |
| |
376 |
| - | |
| 349 | + | |
377 | 350 |
| |
378 | 351 |
| |
379 | 352 |
| |
| |||
382 | 355 |
| |
383 | 356 |
| |
384 | 357 |
| |
| 358 | + | |
| 359 | + | |
| 360 | + | |
| 361 | + | |
| 362 | + | |
| 363 | + | |
| 364 | + | |
| 365 | + | |
| 366 | + | |
| 367 | + | |
| 368 | + | |
| 369 | + | |
| 370 | + | |
385 | 371 |
| |
386 | 372 |
| |
387 | 373 |
| |
| |||
419 | 405 |
| |
420 | 406 |
| |
421 | 407 |
| |
422 |
| - | |
| 408 | + | |
423 | 409 |
| |
424 | 410 |
| |
425 | 411 |
| |
| |||
497 | 483 |
| |
498 | 484 |
| |
499 | 485 |
| |
500 |
| - | |
501 | 486 |
| |
502 | 487 |
| |
503 | 488 |
| |
504 | 489 |
| |
505 | 490 |
| |
506 | 491 |
| |
507 |
| - | |
| 492 | + | |
| 493 | + | |
| 494 | + | |
| 495 | + | |
| 496 | + | |
508 | 497 |
| |
509 | 498 |
| |
510 | 499 |
| |
|
Lines changed: 12 additions & 25 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
9 |
| - | |
| 9 | + | |
10 | 10 |
| |
11 | 11 |
| |
12 | 12 |
| |
| |||
554 | 554 |
| |
555 | 555 |
| |
556 | 556 |
| |
557 |
| - | |
| 557 | + | |
558 | 558 |
| |
559 | 559 |
| |
560 | 560 |
| |
561 |
| - | |
562 |
| - | |
563 |
| - | |
564 |
| - | |
565 |
| - | |
566 |
| - | |
567 |
| - | |
| 561 | + | |
568 | 562 |
| |
569 | 563 |
| |
570 | 564 |
| |
| |||
573 | 567 |
| |
574 | 568 |
| |
575 | 569 |
| |
576 |
| - | |
577 |
| - | |
578 |
| - | |
579 |
| - | |
580 |
| - | |
581 |
| - | |
582 |
| - | |
583 |
| - | |
584 |
| - | |
| 570 | + | |
585 | 571 |
| |
586 | 572 |
| |
587 | 573 |
| |
588 |
| - | |
589 |
| - | |
590 |
| - | |
591 |
| - | |
592 |
| - | |
593 |
| - | |
594 |
| - | |
| 574 | + | |
595 | 575 |
| |
596 | 576 |
| |
597 | 577 |
| |
| |||
958 | 938 |
| |
959 | 939 |
| |
960 | 940 |
| |
| 941 | + | |
| 942 | + | |
| 943 | + | |
| 944 | + | |
| 945 | + | |
| 946 | + | |
| 947 | + | |
961 | 948 |
| |
962 | 949 |
| |
963 | 950 |
| |
|
0 commit comments