Commit 94a88b1
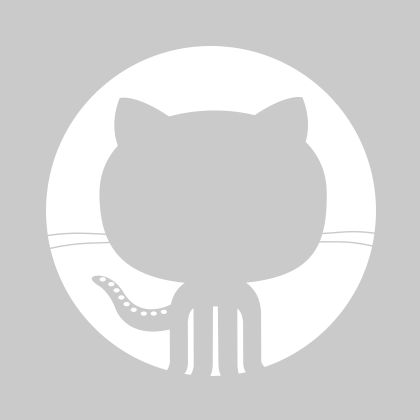
telwertowski
1 parent 7d13075 commit 94a88b1
File tree
12 files changed
+292
-45
lines changed- src
- gui
- plugins
- delimited_text
- georeferencer
- gps_importer
- tools/mapserver_export
12 files changed
+292
-45
lines changedLines changed: 1 addition & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
| 8 | + | |
8 | 9 |
| |
9 | 10 |
| |
10 | 11 |
| |
|
Lines changed: 159 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
| 136 | + | |
| 137 | + | |
| 138 | + | |
| 139 | + | |
| 140 | + | |
| 141 | + | |
| 142 | + | |
| 143 | + | |
| 144 | + | |
| 145 | + | |
| 146 | + | |
| 147 | + | |
| 148 | + | |
| 149 | + | |
| 150 | + | |
| 151 | + | |
| 152 | + | |
| 153 | + | |
| 154 | + | |
| 155 | + | |
| 156 | + | |
| 157 | + | |
| 158 | + | |
| 159 | + |
Lines changed: 52 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + |
Lines changed: 1 addition & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
34 | 34 |
| |
35 | 35 |
| |
36 | 36 |
| |
| 37 | + | |
37 | 38 |
| |
38 | 39 |
| |
39 | 40 |
| |
|
Lines changed: 6 additions & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
216 | 216 |
| |
217 | 217 |
| |
218 | 218 |
| |
219 |
| - | |
| 219 | + | |
220 | 220 |
| |
221 | 221 |
| |
222 | 222 |
| |
| |||
332 | 332 |
| |
333 | 333 |
| |
334 | 334 |
| |
| 335 | + | |
| 336 | + | |
| 337 | + | |
| 338 | + | |
| 339 | + | |
335 | 340 |
| |
336 | 341 |
| |
337 | 342 |
| |
|
Lines changed: 9 additions & 6 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 | 2 |
| |
6 | 3 |
| |
7 | 4 |
| |
| |||
125 | 122 |
| |
126 | 123 |
| |
127 | 124 |
| |
128 |
| - | |
| 125 | + | |
129 | 126 |
| |
130 | 127 |
| |
131 | 128 |
| |
132 | 129 |
| |
133 |
| - | |
| 130 | + | |
134 | 131 |
| |
135 | 132 |
| |
136 | 133 |
| |
| |||
144 | 141 |
| |
145 | 142 |
| |
146 | 143 |
| |
147 |
| - | |
| 144 | + | |
| 145 | + | |
| 146 | + | |
| 147 | + | |
| 148 | + | |
| 149 | + | |
| 150 | + | |
148 | 151 |
| |
149 | 152 |
| |
150 | 153 |
| |
|
Lines changed: 2 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
228 | 228 |
| |
229 | 229 |
| |
230 | 230 |
| |
231 |
| - | |
| 231 | + | |
232 | 232 |
| |
233 | 233 |
| |
234 | 234 |
| |
235 | 235 |
| |
236 | 236 |
| |
237 | 237 |
| |
238 |
| - | |
| 238 | + | |
239 | 239 |
| |
240 | 240 |
| |
241 | 241 |
| |
|
Lines changed: 31 additions & 21 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
61 | 61 |
| |
62 | 62 |
| |
63 | 63 |
| |
64 |
| - | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
65 | 69 |
| |
66 | 70 |
| |
67 | 71 |
| |
| |||
139 | 143 |
| |
140 | 144 |
| |
141 | 145 |
| |
142 |
| - | |
| 146 | + | |
| 147 | + | |
143 | 148 |
| |
144 | 149 |
| |
145 | 150 |
| |
| |||
220 | 225 |
| |
221 | 226 |
| |
222 | 227 |
| |
223 |
| - | |
| 228 | + | |
| 229 | + | |
224 | 230 |
| |
225 | 231 |
| |
226 | 232 |
| |
| |||
232 | 238 |
| |
233 | 239 |
| |
234 | 240 |
| |
235 |
| - | |
236 |
| - | |
237 |
| - | |
238 |
| - | |
239 |
| - | |
240 |
| - | |
241 |
| - | |
242 |
| - | |
243 |
| - | |
244 |
| - | |
245 |
| - | |
246 |
| - | |
247 |
| - | |
248 |
| - | |
249 |
| - | |
250 |
| - | |
251 |
| - | |
| 241 | + | |
| 242 | + | |
| 243 | + | |
| 244 | + | |
| 245 | + | |
| 246 | + | |
| 247 | + | |
| 248 | + | |
| 249 | + | |
| 250 | + | |
| 251 | + | |
| 252 | + | |
| 253 | + | |
| 254 | + | |
| 255 | + | |
| 256 | + | |
| 257 | + | |
| 258 | + | |
| 259 | + | |
| 260 | + | |
252 | 261 |
| |
253 | 262 |
| |
254 | 263 |
| |
| |||
259 | 268 |
| |
260 | 269 |
| |
261 | 270 |
| |
262 |
| - | |
| 271 | + | |
| 272 | + | |
263 | 273 |
| |
264 | 274 |
| |
265 | 275 |
| |
|
Lines changed: 10 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
104 | 104 |
| |
105 | 105 |
| |
106 | 106 |
| |
107 |
| - | |
| 107 | + | |
108 | 108 |
| |
109 | 109 |
| |
110 | 110 |
| |
| |||
192 | 192 |
| |
193 | 193 |
| |
194 | 194 |
| |
195 |
| - | |
| 195 | + | |
196 | 196 |
| |
197 | 197 |
| |
198 | 198 |
| |
| |||
388 | 388 |
| |
389 | 389 |
| |
390 | 390 |
| |
391 |
| - | |
| 391 | + | |
392 | 392 |
| |
393 | 393 |
| |
394 | 394 |
| |
| |||
489 | 489 |
| |
490 | 490 |
| |
491 | 491 |
| |
| 492 | + | |
| 493 | + | |
| 494 | + | |
| 495 | + | |
| 496 | + | |
| 497 | + | |
| 498 | + | |
492 | 499 |
| |
493 | 500 |
| |
494 | 501 |
| |
|
Lines changed: 4 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
| 21 | + | |
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
25 | 25 |
| |
26 | 26 |
| |
| 27 | + | |
27 | 28 |
| |
28 | 29 |
| |
29 |
| - | |
| 30 | + | |
30 | 31 |
| |
31 | 32 |
| |
32 | 33 |
| |
33 | 34 |
| |
34 | 35 |
| |
| 36 | + | |
35 | 37 |
| |
36 | 38 |
| |
37 | 39 |
| |
|
Lines changed: 3 additions & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
39 | 39 |
| |
40 | 40 |
| |
41 | 41 |
| |
42 |
| - | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
43 | 45 |
| |
44 | 46 |
| |
45 | 47 |
| |
|
Lines changed: 14 additions & 9 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
63 | 63 |
| |
64 | 64 |
| |
65 | 65 |
| |
66 |
| - | |
| 66 | + | |
67 | 67 |
| |
68 | 68 |
| |
69 | 69 |
| |
| |||
72 | 72 |
| |
73 | 73 |
| |
74 | 74 |
| |
75 |
| - | |
| 75 | + | |
76 | 76 |
| |
77 | 77 |
| |
78 | 78 |
| |
| |||
103 | 103 |
| |
104 | 104 |
| |
105 | 105 |
| |
106 |
| - | |
| 106 | + | |
107 | 107 |
| |
108 | 108 |
| |
109 | 109 |
| |
110 | 110 |
| |
111 |
| - | |
| 111 | + | |
112 | 112 |
| |
113 | 113 |
| |
114 | 114 |
| |
| |||
139 | 139 |
| |
140 | 140 |
| |
141 | 141 |
| |
142 |
| - | |
| 142 | + | |
143 | 143 |
| |
144 | 144 |
| |
145 | 145 |
| |
146 | 146 |
| |
147 |
| - | |
| 147 | + | |
148 | 148 |
| |
149 | 149 |
| |
150 | 150 |
| |
| |||
366 | 366 |
| |
367 | 367 |
| |
368 | 368 |
| |
369 |
| - | |
| 369 | + | |
370 | 370 |
| |
371 | 371 |
| |
372 | 372 |
| |
373 | 373 |
| |
374 |
| - | |
| 374 | + | |
375 | 375 |
| |
376 | 376 |
| |
377 | 377 |
| |
| |||
390 | 390 |
| |
391 | 391 |
| |
392 | 392 |
| |
393 |
| - | |
| 393 | + | |
394 | 394 |
| |
395 | 395 |
| |
396 | 396 |
| |
| |||
425 | 425 |
| |
426 | 426 |
| |
427 | 427 |
| |
| 428 | + | |
| 429 | + | |
| 430 | + | |
| 431 | + | |
| 432 | + | |
428 | 433 |
| |
429 | 434 |
| |
430 | 435 |
| |
|
0 commit comments